Foundry工具链入门
Foundry工具链入门
到这里我已经成功安装了Foundry(成功获得forge指令)。现在可以用Foundry来初始化一个项目并执行测试。
创建项目
使用 forge init
:
forge init 项目名
注意,这里可能需要先config个代理:
git config --global http.proxy http://127.0.0.1:7890
git config --global https.proxy http://127.0.0.1:7890
之后还是clash开互联网连接,开隧道模式…只能说墙国这辈子有了。注意设完之后,以后所有git操作都要挂隧道,否则会报错说connection reset.
通过默认模板创建的项目结构是:
.
├── lib
├── script
├── src
└── test
和hardhat的差不多。
其余的东西在这里先略过,我目前只需要默认模板。
编写Fork测试
我这里需要测试的代码来自这篇文章,所以我直接从Fork测试开始,略过简单的单机测试。由于之前用的是js进行的测试,而Foundry的测试文件是Counter.t.sol
的格式,所以需要改写一下。
首先看默认模板给出的测试文件:
// SPDX-License-Identifier: UNLICENSED
pragma solidity ^0.8.13;
import {Test, console} from "forge-std/Test.sol";
import {Counter} from "../src/Counter.sol";
contract CounterTest is Test {
Counter public counter;
function setUp() public {
counter = new Counter();
counter.setNumber(0);
}
function test_Increment() public {
counter.increment();
assertEq(counter.number(), 1);
}
function testFuzz_SetNumber(uint256 x) public {
counter.setNumber(x);
assertEq(counter.number(), x);
}
}
这里其实很好理解:在合约中创建要测试的合约实例,之后该怎样就怎样。根据这个规则,改写一下我之前的js。
首先把合约换一下,先import合约类再创建实例:
import {LiquidationOperator} from "../src/LiquidationOperator.sol";
contract CounterTest is Test {
LiquidationOperator public counter;
这种方式比Ethers.js要简单一些,不再需要调合约工厂去拿实例。注意在build之前,要去script里找到Counter.s.sol
改一下里边的内容,在build时默认找的是这个文件:
// SPDX-License-Identifier: UNLICENSED
pragma solidity ^0.8.13;
import {Script, console} from "forge-std/Script.sol";
// 改成自己的文件名和类名
import {LiquidationOperator} from "../src/LiquidationOperator.sol";
contract CounterScript is Script {
LiquidationOperator public counter;
function setUp() public {}
function run() public {
vm.startBroadcast();
counter = new LiquidationOperator(); // 编译
vm.stopBroadcast();
}
}
编译之后先浅浅运行一个简单的测试,在这个测试中我只会fork一下主网并创建合约实例,而不调用任何方法:
contract CounterTest is Test {
LiquidationOperator public counter;
function setUp() public {
counter = new LiquidationOperator();
}
// 这个方法是我加到合约里的,里边没有逻辑
function test_foundry_test() public {
counter.foundry_test();
}
}
可以看出,即使我只想创建一个合约对象,但是测试文件仍需包含两个函数。执行测试时foundry会去找test_
开头的函数,如果没有写的话会报错。写好后进行fork测试:
forge test --fork-url https://eth.merkle.io --fork-block-number 12489619
有一些格式警告但是通过。这就意味着该合约已经能够被部署在我要求的环境下,而且constructor也运转正常。
ok,现在可以测试一下清算逻辑。foundry默认是不会打印任何日志信息的,为了打印出日志,需要在测试命令前加上-vvvv
参数:
forge test -vvvv --fork-url https://eth.merkle.io --fork-block-number 12489619
这个测试失败了。好在打印了log,可以清晰看出是哪里出了问题:
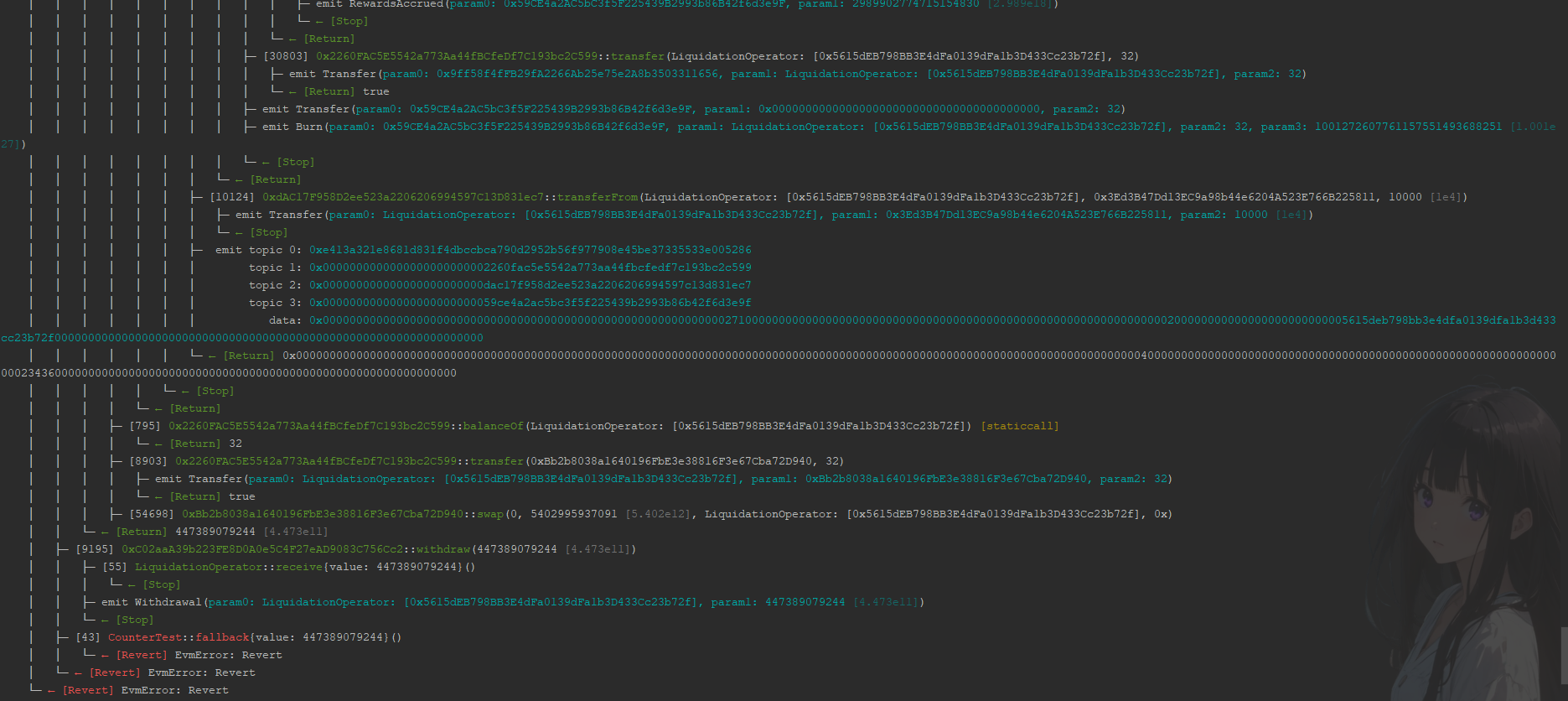
可以看出,内层的交易失败,连带着外边像剥洋葱似的一层一层都会revert。
这里也略过我重写测试合约的过程,直接贴一个正确的代码:
// SPDX-License-Identifier: UNLICENSED
pragma solidity ^0.8.13;
import {Test, console} from "forge-std/Test.sol";
import {LiquidationOperator} from "../src/LiquidationOperator.sol";
contract CounterTest is Test {
LiquidationOperator public liquidationOperator;
address public liquidator;
function setUp() public {
resetFork();
}
function resetFork() internal {
vm.createSelectFork("https://eth.merkle.io", 12489619);
liquidator = vm.addr(1);
vm.startPrank(liquidator);
liquidationOperator = new LiquidationOperator();
vm.stopPrank();
}
function testLiquidation() public {
resetFork();
vm.startPrank(liquidator);
uint256 beforeBalance = liquidator.balance;
vm.fee(0);
liquidationOperator.operate();
uint256 afterBalance = liquidator.balance;
uint256 profit = afterBalance - beforeBalance;
console.log("Profit (wei): ", profit);
assertGt(profit, 0, "not profitable");
vm.stopPrank();
}
}
在setUp之后,测试代码会直接执行resetFork()
,它能确保每次测试都新fork一遍。如果不这样可能会导致只有第一次有正确的收益(因为第一次清算完后边能清算的就会少)。这样一来,执行test时也就不需要那些fork参数了:
forge test -vvv
之后就是核心的测试逻辑,可以看出它比Ethers.js
版本的简约很多,这里不赘述他的意思。但是有一个要注意的点,solidity中的console.log
并非什么都能打印,它不能打印小数,如果强行把profit除以1e18的话会自动四舍五入。如果要打印小数。需要手动把小数转换成一个string才能作为log的参数,否则报错。